HTML5 Game Development Tutorial 27 - Canvas buttons and start screen
HTML5 Game Development Tutorial 27 - Canvas buttons and start screen
Viewing SVG Files
Viewing SVG Files:
Most of the web browsers can display SVG just like they can display PNG, GIF, and JPG. Internet Explorer users may have to install the Adobe SVG Viewer to be able to view SVG in the browser.
Embeding SVG in HTML5
HTML5 allows embeding SVG directly using <svg>...</svg> tag which has following simple syntax:
<svg xmlns="http://www.w3.org/2000/svg"> ... </svg> |
Firefox 3.7 has also introduced a configuration option ("about:config") where you can enable HTML5 using the following steps:
- Type about:config in your Firefox address bar.
- Click the "I'll be careful, I promise!" button on the warning message that appears (and make sure you adhere to it!).
- Type html5.enable into the filter bar at the top of the page.
- Currently it would be disabled, so click it to toggle the value to true.
Now your Firefox HTML5 parser should now be enabled and you should be able to experiment with the following examples.
HTML5 - SVG Circle
Following is the HTML5 version of an SVG example which would draw a cricle using <circle> tag:
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Circle</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <circle id="redcircle" cx="50" cy="50" r="50" fill="red" /> </svg> </body> </html> |
This would produce following result in HTML5 enabled latest version of Firefox.
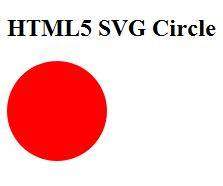
To learn this concept - Do Online Practice using Firefox 3.7 or higher version.
HTML5 - SVG Rectangle
Following is the HTML5 version of an SVG example which would draw a rectangle using <rect> tag:
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Rectangle</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <rect id="redrect" width="300" height="100" fill="red" /> </svg> </body> </html> |
This would produce following result in HTML5 enabled latest version of Firefox.

To learn this concept - Do Online Practice using Firefox 3.7 or higher version.
HTML5 - SVG Line
Following is the HTML5 version of an SVG example which would draw a line using <line> tag:
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Line</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <line x1="0" y1="0" x2="200" y2="100" style="stroke:red;stroke-width:2"/> </svg> </body> </html> |
You can use style attribute which allows you to set additional style information like stroke and fill colors, width of the stroke etc.
This would produce following result in HTML5 enabled latest version of Firefox.
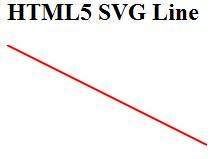
To learn this concept - Do Online Practice using Firefox 3.7 or higher version.
HTML5 - SVG Ellipse
Following is the HTML5 version of an SVG example which would draw an ellipse using <ellipse> tag:
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Ellipse</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <ellipse cx="100" cy="50" rx="100" ry="50" fill="red" /> </svg> </body> </html> |
This would produce following result in HTML5 enabled latest version of Firefox.
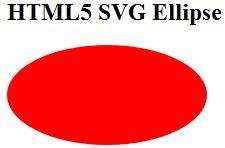
To learn this concept - Do Online Practice using Firefox 3.7 or higher version.
HTML5 - SVG Polygon
Following is the HTML5 version of an SVG example which would draw a polygon using <polygon> tag:
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Polygon</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <polygon points="20,10 300,20, 170,50" fill="red" /> </svg> </body> </html> |
This would produce following result in HTML5 enabled latest version of Firefox.

To learn this concept - Do Online Practice using Firefox 3.7 or higher version.
HTML5 - SVG Polyline
Following is the HTML5 version of an SVG example which would draw a polyline using <polyline> tag:
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Polyline</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <polyline points="0,0 0,20 20,20 20,40 40,40 40,60" fill="red" /> </svg> </body> </html> |
This would produce following result in HTML5 enabled latest version of Firefox.
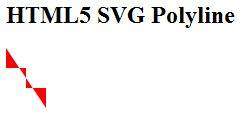
To learn this concept - Do Online Practice using Firefox 3.7 or higher version.
HTML5 - SVG Gradients
Following is the HTML5 version of an SVG example which would draw a ellipse using <ellipse> tag and would use <radialGradient> tag to define an SVG radial gradient.
Similar way you can use <linearGradient> tag to create SVG linear gradient.
<!DOCTYPE html> <head> <title>SVG</title> <meta charset="utf-8" /> </head> <body> <h2>HTML5 SVG Gradient Ellipse</h2> <svg id="svgelem" height="200" xmlns="http://www.w3.org/2000/svg"> <defs> <radialGradient id="gradient" cx="50%" cy="50%" r="50%" fx="50%" fy="50%"> <stop offset="0%" style="stop-color:rgb(200,200,200); stop-opacity:0"/> <stop offset="100%" style="stop-color:rgb(0,0,255); stop-opacity:1"/> </radialGradient> </defs> <ellipse cx="100" cy="50" rx="100" ry="50" style="fill:url(#gradient)" /> </svg> </body> </html> |
This would produce following result in HTML5 enabled latest version of Firefox.
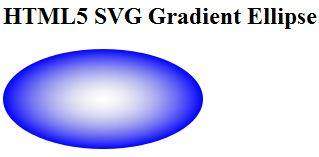
The head tag
The head tag

- Web sites have two audiences:
- The first is human
- The other "spiders", "bots" or "web crawlers"
While the contents of the head tag displays little to human viewers it's important to the bots. Search engines have crawlers (e.g. Google's is called "Googlebot") that constantly surf the net following links from one site to another collecting and indexing everything they find. Google has an algorithm that then decides which pages are most relevant to search terms.
Web sites that read well to spiders are most likely to appear high in search engine results. This is what is called SEO or Search Engine Optimization. Books – big thick books – have been written on SEO. We don't have the time to go into it in great depth, but I will repeatedly touch on it as you proceed through the tutorial.
The single most important thing you can do for SEO is write a good title tag. My title tag for this page is:
<title>
Head tag - HTML5 tutorial</title>
. It doesn't appear on the page itself but does show in the bar across the top of the browser and in the button in the taskbar, usually found at the bottom of the monitor on a PC.
The title also appears in Google's search results. For example:

Try to make it keyword heavy, but don't forget that people will also be reading it. Ideally the title should be less than 64 characters in length.
The second most important thing you can do is be realistic. At this point it's all but impossible to get high ranking on popular search words. Jimmy's Flower Shop isn't going to show up when someone searches "Flowers". However google "Jimmy's Flower Shop" or "Ogden Florist" and up comes www.jimmysflowers.com. If some guy's wife in Ogden is really ticked off, Jimmy just made a sale.
Jimmy's web site makes Jimmy money in part because whoever made Jimmy's site took the time to carefully chose the proper keywords and to write a good title. It is astonishing how few web designers take these two simple but crucial steps.
Meta tags also go in the head tag. Two in particular are important; both of which you can see in this page's source code.
- The charset meta tag:
<meta charset="utf-8">
- The description meta tag:
<meta name="description" content="The head tag contains the title and meta tags - important to the search engines, and information for the browser to properly display the page.">
.
The former tells the browser what type of letters the page is written in. If your web site is in a language other than English be careful to use the correct charset. If your page is in plain old vanilla English just copy and paste
<meta charset="UTF-8">
just above the title*, and with any luck you'll never have to think about it again.
The latter sometimes shows up in search results and it can help in SEO.
There is also a "keyword" meta tag originally created to help in SEO but has become essentially worthless because so many web designers cheated. In the late '90s searching "dentist in Denver" might lead you to "porno in Portland". If you weren't careful you never would get your teeth fixed.
Tag - html
Tag - html
Now we go to our first "tag". *

With a couple of important exceptions (which we will get into later) all tags have to be opened and closed. Take a look at the code to the right. The opening tag is:
<html>
. The closing tag is </html>
– the difference being the "/" in the closing tag.
Tags are containers. The html tag indicates that everything between
<html>
and</html>
is code that conforms to the standards of the type of HTML dictated by the doctype declaration – in this case HTML5.
Everything between the opening tag and closing tag are inside that tag and therefore have the attributes that tag gives them. Those attributes can be modified. For example in this site I have changed the default color (black) for the
</html>
tag to dark blue.- Inside the
<html>
tag are two other important tags: - the
<head>
tag - the
<body>
tag
First let's take a look at the
<head>
tag.HTML5 – A tutorial for beginners
HTML5 – A tutorial for beginners
It's not rocket science.

To the uninitiated HTML5 might look like rocket science, but it's not. Believe me, if you passed high school algebra you can figure this out.
My goal on html-5-tutorial.com is to give you a good solid start on doing just that.
You won't be ready to quit your day job, but...
...you have to start somewhere!
HTML-5-Tutorial.com is geared to those who want to edit and write HTML code by hand. Knowing how the nuts and bolts of a webpage fit and work together gives you the means to utilize HTML5's full potential. Debugging and tweaking the code is easier and it greatly facilitates search engine optimization (SEO).
So what is HTML5? HTML5 is a language – a language computers use to "speak" to each other. However, left to their own devices, computers are mute machines – we aren't, and in the words of the Great Bard...
The State of HTML5 Games in Asia - New Game 2011
Game Development With HTML5